Overview of the This Day In History Navigation
Screens
There will be two paths in our app. The onboarding path and the main path.
The onboarding path will consist of the following screens:
- Welcome: A welcome message in the language of the device.
- Language: Allows to pick one of the 8 languages the application content can be displayed in.
The main path will include the following screens:
- Home: A list view of historical events, presenting a succint information about an event in each row.
- Detail: An expanded view of the event, along with a carousel of associated events.
- About: What our app is about and how great it is.
- Language: Choose any other language the app supports at any time.
- Settings: Switch between light and dark modes.
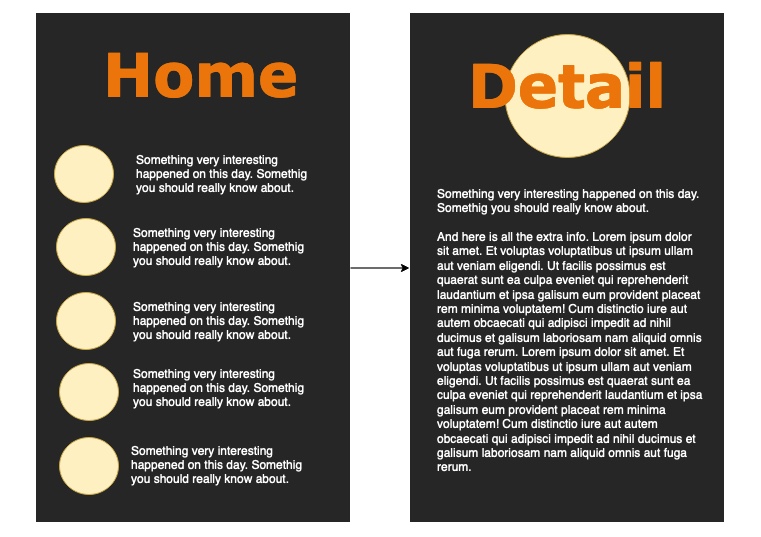
The following three screens will be selectable from the navigation drawer. Note that the Languages screen appears here again, even though it was part of the onboarding path. We don't want to have the user locked in a single pre-selected language - they should be able to switch languages any time they want. The About screen is pretty obvious - we praise our app; and the Settings screen will allow to switch themes.
The Navigation Component
The Navigation component has three main parts:- NavController: Responsible for navigating between destinations.
- NavHost: Composable acting as a container for displaying the current destination of the NavGraph.
- NavGraph: Maps composable destinations to navigate to.
The first navigation component we need to implement is the NavGraph. In the classic view system, navigation graph is expressed in xml and Android Studio provides a visual editor to help with producing the xml file. But we are in the Compose world, and will be using Kotlin code for everything (ok, almost everything). In This Day In History app, we need to have two graphs - one for the onboarding workflow, and the other for the main workflow. We will have six navigable composables altogether.
enum class NavRoutes {
IntroRoute,
MainRoute
}
enum class MainNavOption {
HistoryScreen,
DetailScreen,
LanguagesScreen,
ThemeScreen,
AboutScreen
}
enum class IntroNavOption {
WelcomeScreen,
LanguagesScreen
}
fun NavGraphBuilder.introGraph(navController: NavController) {
navigation(startDestination = IntroNavOption.WelcomeScreen.name, route = NavRoutes.IntroRoute.name) {
composable(IntroNavOption.WelcomeScreen.name){
WelcomeScreen()
}
composable(IntroNavOption.LanguagesScreen.name){
LanguageScreen()
}
}
}
fun NavGraphBuilder.mainGraph(
navController: NavController
) {
navigation(startDestination = MainNavOption.HistoryScreen.name, route = NavRoutes.MainRoute.name) {
composable(MainNavOption.HistoryScreen.name) {
//todo History Screen Goes here
}
composable(
MainNavOption.DetailScreen.name
)
{ backStackEntry ->
//todo Detail Screen goes here
}
composable(
MainNavOption.LanguagesScreen.name
) {
//todo Language Screen goes here
}
composable(
MainNavOption.ThemeScreen.name
) {
//todo Theme Screen
}
composable(
MainNavOption.AboutScreen.name
) {
//todo About Screen
}
}
}