This Day In History Jetpack Compose App - Logo Composable
Objective:
Build our Logo Composable and place it in the Navigation Drawer (later on, it will be used in other screens too). At the end of this exercise, the Navigaton Drawer will look like below. It will require more work to style it correctly, but that will be done later. We will take care of the functionaly first.
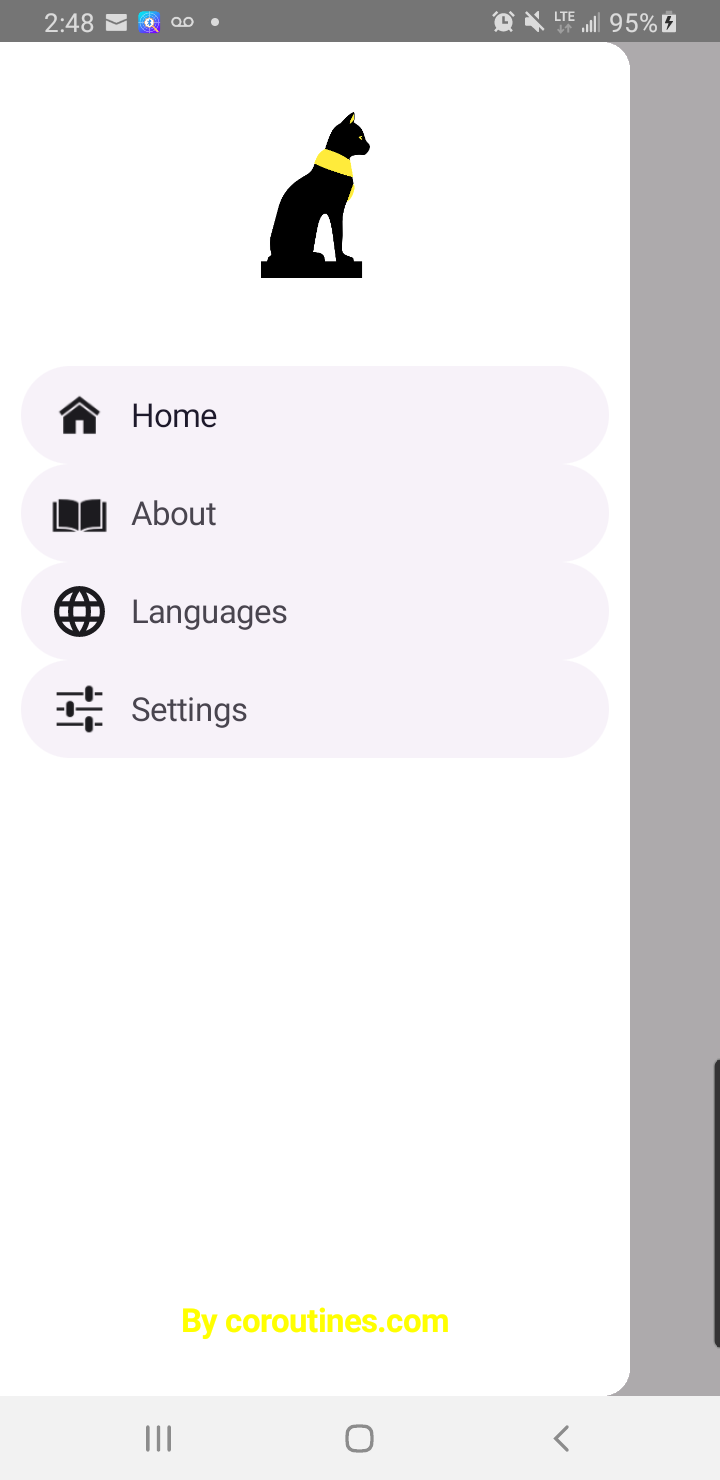
ISettingsViewModel and its mock implementation: SettingsViewModelMock
First, we need to introduce a mock version of the SettingsViewModel. Previously, we defined the Settings interface to be able to:
- Retrieve AppConfigurationState (based on the MVI pattern).
- Set Application Theme: This will be done from the Theme Screen.
- Set Device Language: This will be done on app start-up automatically.
- Set Onboarded Status: This will be done once, when the user has gone thru the onboarding route on the first app start-up.
- Set App Language: This will be done from the Languages Screen, during onboarding first, and at any time during application usage, as we want to support in-app language selection
interface ISettingsViewModel {
val appConfigurationState: StateFlow<AppConfigurationState>
fun setAppTheme(theme: ThisDayInHistoryThemeEnum)
fun setAppLanguage(langEnum: LangEnum)
fun setDeviceLanguage(language: String)
fun setOnboarded()
val aboutDescription: Flow<String>
}
And Configuration State was defined as below:
data class AppConfigurationState(
val isLoading: Boolean,
val isOnboarded: Boolean,
val useDynamicColors: Boolean,
val appTheme: ThisDayInHistoryThemeEnum,
val appLanguage: LangEnum,
val deviceLanguage: String
)
Let us now create the mock version of the SettingsViewModel. Why mock? I prefer to test the concept with mock data first, before developing real services. The mock version will also come handy when we get to the unit testing.
package com.coroutines.thisdayinhistory.ui.viewmodels
import androidx.lifecycle.ViewModel
import androidx.lifecycle.viewModelScope
import com.coroutines.data.models.LangEnum
import com.coroutines.data.models.Languages
import com.coroutines.thisdayinhistory.ui.state.AppConfigurationState
import com.coroutines.thisdayinhistory.ui.theme.ThisDayInHistoryThemeEnum
import kotlinx.coroutines.flow.MutableStateFlow
import kotlinx.coroutines.flow.SharingStarted
import kotlinx.coroutines.flow.StateFlow
import kotlinx.coroutines.flow.map
import kotlinx.coroutines.flow.stateIn
import kotlinx.coroutines.flow.update
private data class SettingsViewModelStateMock(
val isLoading: Boolean = true,
val isOnboarded: Boolean = false,
val useDynamicColors: Boolean = true,
val appTheme: ThisDayInHistoryThemeEnum = ThisDayInHistoryThemeEnum.Dark,
val appLanguage: LangEnum = LangEnum.ENGLISH,
val deviceLanguage: String = ""
) {
fun asActivityState() = AppConfigurationState(
isLoading = isLoading,
isOnboarded = isOnboarded,
useDynamicColors = useDynamicColors,
appTheme = appTheme,
appLanguage = appLanguage,
deviceLanguage = deviceLanguage
)
}
class SettingsViewModelMock(
historyCatThemeEnum: ThisDayInHistoryThemeEnum = ThisDayInHistoryThemeEnum.Auto
) : ViewModel(), ISettingsViewModel {
private var _aboutDescription = MutableStateFlow(Languages.ENGLISH.appDescription)
private val viewModelState = MutableStateFlow(value = SettingsViewModelStateMock())
override val appConfigurationState = viewModelState
.map { it.asActivityState().copy(appTheme = historyCatThemeEnum) }.stateIn(
scope = viewModelScope,
started = SharingStarted.WhileSubscribed(stopTimeoutMillis = 5_000),
initialValue = viewModelState.value.asActivityState()
)
override fun setAppTheme(theme: ThisDayInHistoryThemeEnum) {
viewModelState.update { state ->
state.copy(
appTheme = state.appTheme
)
}
}
override fun setAppLanguage(langEnum: LangEnum) {
viewModelState.update { state ->
state.copy(
appLanguage = langEnum
)
}
}
override fun setDeviceLanguage(language: String) {
TODO("Not yet implemented")
}
override fun setOnboarded() {
TODO("Not yet implemented")
}
override val aboutDescription: StateFlow<String>
get() = _aboutDescription
}
package com.coroutines.thisdayinhistory.ui.constants
const val CAT_LOGO_HEADER_TEXT_TAG = "catLogoHeaderText"
package com.coroutines.thisdayinhistory.ui.previewProviders
import androidx.compose.ui.tooling.preview.PreviewParameterProvider
import com.coroutines.data.models.LangEnum
import com.coroutines.thisdayinhistory.ui.state.AppConfigurationState
import com.coroutines.thisdayinhistory.ui.theme.ThisDayInHistoryThemeEnum
class AppConfigurationStateProvider : PreviewParameterProvider {
override val values = listOf(
AppConfigurationState(
isLoading = false,
isOnboarded = true,
useDynamicColors = false,
appTheme = ThisDayInHistoryThemeEnum.Dark,
appLanguage = LangEnum.ENGLISH,
deviceLanguage = "en"),
AppConfigurationState(
isLoading = false,
isOnboarded = true,
useDynamicColors = false,
appTheme = ThisDayInHistoryThemeEnum.Light,
appLanguage = LangEnum.ENGLISH,
deviceLanguage = "en"),
AppConfigurationState(
isLoading = true,
isOnboarded = true,
useDynamicColors = false,
appTheme = ThisDayInHistoryThemeEnum.Dark,
appLanguage = LangEnum.ENGLISH,
deviceLanguage = "en"),
AppConfigurationState(
isLoading = true,
isOnboarded = true,
useDynamicColors = false,
appTheme = ThisDayInHistoryThemeEnum.Light,
appLanguage = LangEnum.ENGLISH,
deviceLanguage = "en"),
).asSequence()
}
package com.coroutines.thisdayinhistory.ui.components
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Spacer
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.height
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.layout.ContentScale
import androidx.compose.ui.platform.testTag
import androidx.compose.ui.text.style.TextAlign
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.tooling.preview.PreviewParameter
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
import coil.compose.rememberAsyncImagePainter
import coil.request.ImageRequest
import com.coroutines.thisdayinhistory.R
import com.coroutines.thisdayinhistory.ui.state.AppConfigurationState
import com.coroutines.thisdayinhistory.ui.theme.ThisDayInHistoryTheme
import com.coroutines.thisdayinhistory.ui.theme.ThisDayInHistoryThemeEnum
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.layout.size
import androidx.compose.ui.platform.LocalContext
import com.coroutines.thisdayinhistory.ui.constants.CAT_LOGO_HEADER_TEXT_TAG
import com.coroutines.thisdayinhistory.ui.previewProviders.AppConfigurationStateProvider
import com.coroutines.thisdayinhistory.ui.viewmodels.SettingsViewModelMock
@Composable
fun CatLogo(settings: AppConfigurationState) {
val catLogoDrawable =
if (settings.appTheme == ThisDayInHistoryThemeEnum.Dark) R.drawable.cat_logo_for_dark_theme
else R.drawable.cat_logo_for_light_theme
Image(
painter = rememberAsyncImagePainter(
ImageRequest.Builder(LocalContext.current)
.placeholder(catLogoDrawable)
.data(data = catLogoDrawable)
.apply(block = fun ImageRequest.Builder.() {
crossfade(true)
}).build()
),
contentDescription = null,
contentScale = ContentScale.Inside,
modifier = Modifier
.testTag(catLogoDrawable.toString())
.fillMaxWidth()
.size(175.dp)
.padding(40.dp)
)
Spacer(
modifier = Modifier
.fillMaxWidth()
.height(5.dp)
)
Text(
"History Cat",
modifier = Modifier.testTag(CAT_LOGO_HEADER_TEXT_TAG),
color = MaterialTheme.colorScheme.onBackground,
textAlign = TextAlign.Center,
lineHeight = 20.sp,
style = MaterialTheme.typography.headlineSmall,
)
Spacer(
modifier = Modifier
.fillMaxWidth()
.height(5.dp)
)
}
@Composable
@Preview()
fun CatLogoPreview(
@PreviewParameter(
AppConfigurationStateProvider::class,
limit = 2) appConfigurationState: AppConfigurationState
) {
val settingsViewModel = SettingsViewModelMock(appConfigurationState.appTheme)
ThisDayInHistoryTheme(
settingsViewModel
) {
val appThemeColor = MaterialTheme.colorScheme.background
Surface(
modifier = Modifier.background(appThemeColor)
) {
CatLogo(settings = appConfigurationState)
}
}
}
package com.coroutines.thisdayinhistory.drawer
import android.annotation.SuppressLint
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.Spacer
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.height
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.DrawerValue
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.ModalDrawerSheet
import androidx.compose.material3.ModalNavigationDrawer
import androidx.compose.material3.Scaffold
import androidx.compose.material3.Text
import androidx.compose.material3.TopAppBarDefaults
import androidx.compose.material3.rememberDrawerState
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.rememberCoroutineScope
import androidx.compose.runtime.setValue
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Brush
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.input.nestedscroll.nestedScroll
import androidx.compose.ui.text.font.FontWeight
import androidx.compose.ui.text.style.TextAlign
import androidx.compose.ui.unit.dp
import androidx.lifecycle.compose.collectAsStateWithLifecycle
import androidx.navigation.NavController
import androidx.navigation.compose.currentBackStackEntryAsState
import com.coroutines.thisdayinhistory.ui.components.CatLogo
import com.coroutines.thisdayinhistory.ui.viewmodels.ISettingsViewModel
@OptIn( ExperimentalMaterial3Api::class)
@Composable
fun AppNavigationDrawerWithContent(
navController: NavController,
settingsViewModel: ISettingsViewModel,
content: @Composable () -> Unit
) {
val settingsViewModelState by settingsViewModel.appConfigurationState.collectAsStateWithLifecycle()
val items = navDrawerItems()
val drawerState = rememberDrawerState(DrawerValue.Closed)
val scope = rememberCoroutineScope()
val selectedItem = remember { mutableStateOf(items[0]) }
var isItemImageExpanded by remember { mutableStateOf(false) }
ModalNavigationDrawer(
drawerState = drawerState,
drawerContent = {
ModalDrawerSheet {
Column (
Modifier
.fillMaxSize()
.background(
brush = Brush.verticalGradient(
colors = mutableListOf(
Color.White,
Color.White
)
)
))
{
CatLogo(settings = settingsViewModelState )
Spacer(
modifier = Modifier
.fillMaxWidth()
.height(5.dp)
)
val navBackStackEntry by navController.currentBackStackEntryAsState()
val currentRoute = navBackStackEntry?.destination?.route
items.forEach { item ->
BuildNavigationDrawerItem(
item,
currentRoute,
scope,
drawerState,
selectedItem,
navController
)
}
Spacer(modifier = Modifier.weight(1f))
Text(
text = "By coroutines.com",
color = Color.Yellow,
textAlign = TextAlign.Center,
fontWeight = FontWeight.Bold,
modifier = Modifier
.padding(12.dp)
.padding(bottom = 20.dp)
.align(Alignment.CenterHorizontally)
)
}
}
}
) {
val scrollBehavior = TopAppBarDefaults.enterAlwaysScrollBehavior()
Scaffold(
modifier = Modifier.nestedScroll(scrollBehavior.nestedScrollConnection ),
topBar = {
//todo
},
bottomBar = {
//todo
}
) { paddingValues ->
Column(
Modifier
.fillMaxSize()
.padding(top = paddingValues.calculateTopPadding())
) {
content()
}
}
}
}
@Composable
fun MainContent(settingsViewModel: ISettingsViewModel,){
val navController = rememberNavController()
AppNavHost(
navController = navController,
settingsViewModel = settingsViewModel,
isOnboarded = true
)
}
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
runUi()
}
private fun runUi() = setContent {
val settingsViewModel: ISettingsViewModel = SettingsViewModelMock()
MainContent(settingsViewModel)
}
}
FATAL EXCEPTION: main Process: com.coroutines.thisdayinhistory, PID: 26493 java.lang.IllegalStateException: CompositionLocal LocalLifecycleOwner not present at androidx.lifecycle.compose.LocalLifecycleOwnerKt$LocalLifecycleOwner$1.invoke(LocalLifecycleOwner.kt:26) at androidx.lifecycle.compose.LocalLifecycleOwnerKt$LocalLifecycleOwner$1.invoke(LocalLifecycleOwner.kt:25) at kotlin.SynchronizedLazyImpl.getValue(LazyJVM.kt:74) at androidx.compose.runtime.LazyValueHolder.getValue(ValueHolders.kt:31) at androidx.compose.runtime.CompositionLocalMapKt.read(CompositionLocalMap.kt:90) at androidx.compose.runtime.ComposerImpl.consume(Composer.kt:2135)
[versions]
lifecycleRuntimeCompose = "2.7.0"
#lifecycleRuntimeComposeAndroid = "2.8.0"
[libraries]
#androidx-lifecycle-runtime-compose-android = { group = "androidx.lifecycle", name = "lifecycle-runtime-compose-android", version.ref = "lifecycleRuntimeComposeAndroid" }
androidx-lifecycle-runtime-compose = { module = "androidx.lifecycle:lifecycle-runtime-compose", version.ref = "lifecycleRuntimeCompose" }
and then in the app module gradle file:
dependencies {
//...
implementation(libs.androidx.lifecycle.runtime.compose)
//...
}