This Day In History Jetpack Compose App - About Screen
Objective:
Build our About Screen and connect it to a mock SettingsViewModel. At the end of this exercise, the About Screen will look like below. It will require more work to style it correctly, but that will be done later. We will take care of the functionality first.
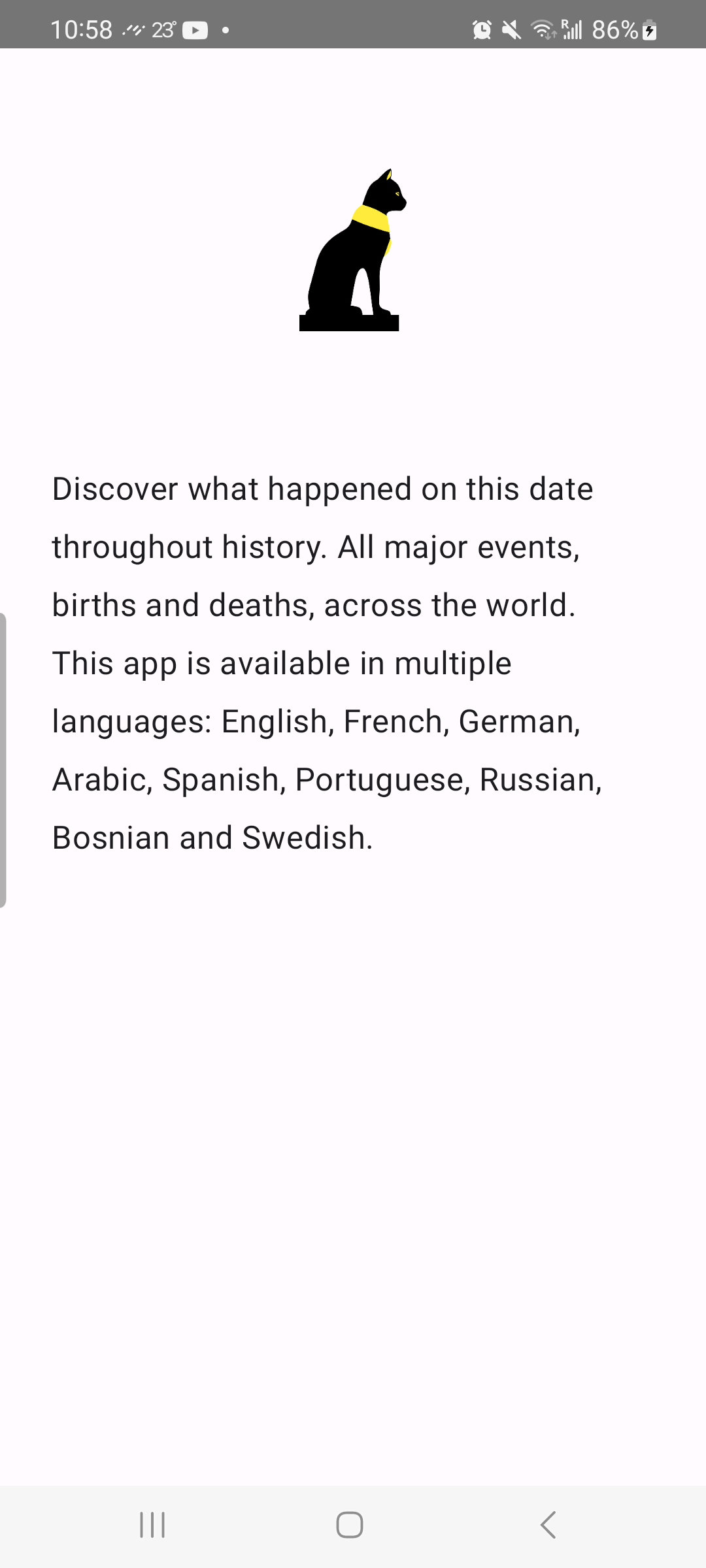
About Screen functionalities
The about screen will do the following:
- Display App Logo at the top.
- Display App Description in the in-app chosen language.
Code
Tag Constants
Add the following constants to the ui.constants package. We will need them for unit tests.
package com.coroutines.thisdayinhistory.ui.constants
//add two new entries:
const val ABOUT_SCREEN_COLUMN_TAG = "aboutScreenColumn"
const val ABOUT_SCREEN_TEXT_TAG = "aboutScreenText"
Preview Providers
And LanguageEnumProvider to the ui.previewProviders package.
package com.coroutines.thisdayinhistory.ui.previewProviders;
import androidx.compose.ui.tooling.preview.PreviewParameterProvider
import com.coroutines.data.models.LangEnum
class LanguageEnumProvider : PreviewParameterProvider<LangEnum> {
override val values = listOf(
LangEnum.ENGLISH,
LangEnum.SWEDISH,
LangEnum.PORTUGUESE,
LangEnum.SPANISH,
LangEnum.RUSSIAN,
LangEnum.FRENCH,
LangEnum.GERMAN,
LangEnum.ARABIC).asSequence()
}
About Screen Composable
And now the About Screen Composable itself, together with the preview function.
package com.coroutines.thisdayinhistory.ui.viewmodels
@Composable
fun AboutScreen(
modifier: Modifier = Modifier,
viewModel: ISettingsViewModel = SettingsViewModelMock()
) {
val about = Languages.from(
viewModel.appConfigurationState.value.appLanguage.langId)?.appDescription
?: Languages.ENGLISH.appDescription
val settings by viewModel.appConfigurationState.collectAsStateWithLifecycle()
Column(
modifier = modifier
.testTag(ABOUT_SCREEN_COLUMN_TAG)
.fillMaxSize()
.background(MaterialTheme.colorScheme.background),
horizontalAlignment = Alignment.CenterHorizontally
) {
Spacer(modifier = Modifier.height(30.dp))
CatLogo(settings)
Spacer(modifier = Modifier.height(20.dp))
Text(
text = about,
modifier = Modifier
.testTag(ABOUT_SCREEN_TEXT_TAG)
.padding(30.dp, 10.dp)
.verticalScroll(rememberScrollState()),
color = MaterialTheme.colorScheme.onBackground,
lineHeight = 25.sp,
style = MaterialTheme.typography.bodyMedium,
)
}
}
@Composable
@Preview()
fun AboutScreenPreview(@PreviewParameter(LanguageEnumProvider::class, limit = 11) langEnum: LangEnum) {
val settingsViewModel = SettingsViewModelMock(ThisDayInHistoryThemeEnum.Dark)
settingsViewModel.setAppLanguage(langEnum)
ThisDayInHistoryTheme(
settingsViewModel
) {
val appThemeColor = MaterialTheme.colorScheme.background
Surface(
modifier = Modifier.background(appThemeColor)
) {
AboutScreen(
viewModel = settingsViewModel,
)
}
}
}